type
status
date
slug
summary
tags
category
icon
password
AI summary
在前一篇文章分析MySQL连接问题的时候,顺带学习了一下连接建立的过程。
首先,在MySQL客户端和服务端之间的TCP连接建立之后,服务端会立马发起
Handshake
流程。此时发起的包在Wireshark上显示为Server Greeting
包,而MySQL官方文档里叫做Handshake
包。这个包是服务端用来告知客户端自己的SSL/TLS版本信息、自己支持的能力(比如有没有设置SSL/TLS相关的配置),并且会尝试乐观猜测一下客户端要用的身份认证插件(Authentication Plugin
),也就是Server里配置的default_authentication_plugin
(较新的版本可能还和authentication_policy
有关)客户端接收到这个Handshake包之后,会结合自己支持的能力,来判断后续应该走什么流程:
- 如果服务端配置了
require_secure_transport = ON
,那么客户端必须使用加密连接。如果客户端未开启SSL/TLS,则连接会报错
- 如果服务端未配置
require_secure_transport = ON
,那么启用不启用主要看客户端。在mysql-connector-java-5.1.48
版本中,如果服务端是5.7
以上的版本并且配置了SSL/TLS,那么客户端默认就会启用加密连接
普通连接
下面我们先看看不启用加密连接的情况。收到Handshake包之后,客户端开始尝试登录(去服务端验证身份),也就是会在Handshake Response里携带身份认证的信息。MySQL对于验证身份采用了插件化的思想,支持不同的
AuthenticationPlugin
。比如常见的mysql_native_password
、caching_sha2_password
、sha256_password
。不同的AuthenticationPlugin
在服务端和客户端端都有对应的实现逻辑。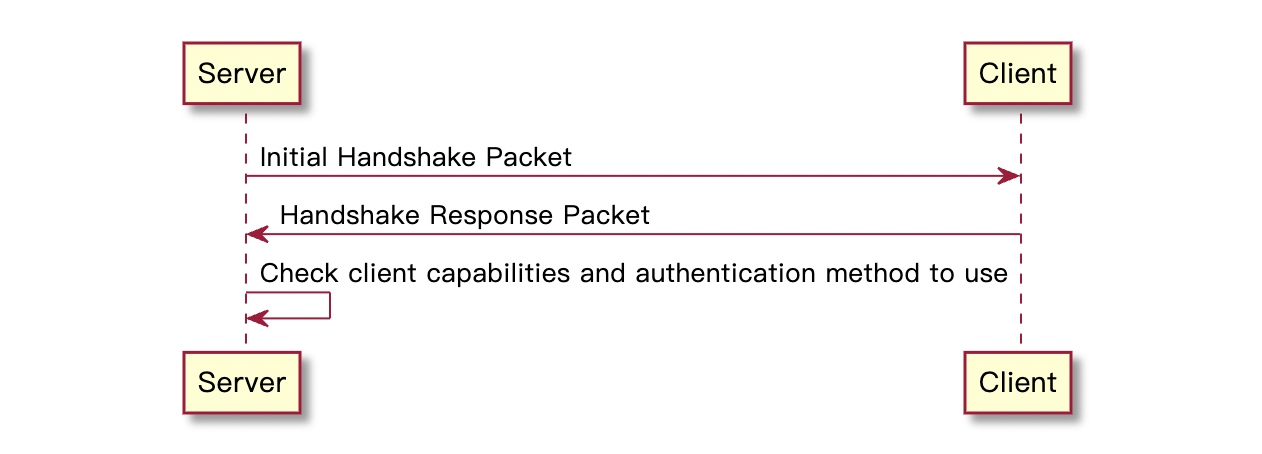
客户端确定Authentication Plugin
那么客户端此时如何确定用哪个身份认证插件去响应服务端呢?从
mysql-connector-java-5.1.48
来看,一般是用服务端下发的,除非一些特殊情况。通过上面的代码,首先可以看到客户端对于身份认证的处理是多轮的,需要和服务端协商确定:
- 优先使用服务端在Handshake包里下发的
authentication_plugin
。
- 如果下发的插件客户端找不到,那么会使用客户端配置的默认插件
还有一种特殊情况,就是如果服务端下发的是
sha256_password
,但是在当前的配置条件下客户端无法获得服务端的RSA公钥,这种情况也会使用客户端配置的默认插件。但是如果此时客户端配置的不是sha256_password
,此次请求不会设置密码,防止有安全风险,看注释的意思是会等后续服务端会下发Auth Switch切换认证方式,再发送密码进行身份验证。但是我很好奇,为什么只有sha256_password
有这个优化链路,caching_sha2_password
也需要RSA公钥啊,为什么没有这条优化链路?Auth Switch
刚才提到的Auth Switch是身份验证过程中的一个特殊流程,就是当服务端发现客户端选择的插件和服务端为该用户配置的认证插件不一致的时候,会发起一个Auth Switch的流程,让客户端切换身份认证。也就是说,服务端会以
mysql.user
表里配置的插件为准,当客户端提交一个不一样的身份认证插件上来,会被服务端驳回并切换到正确的插件。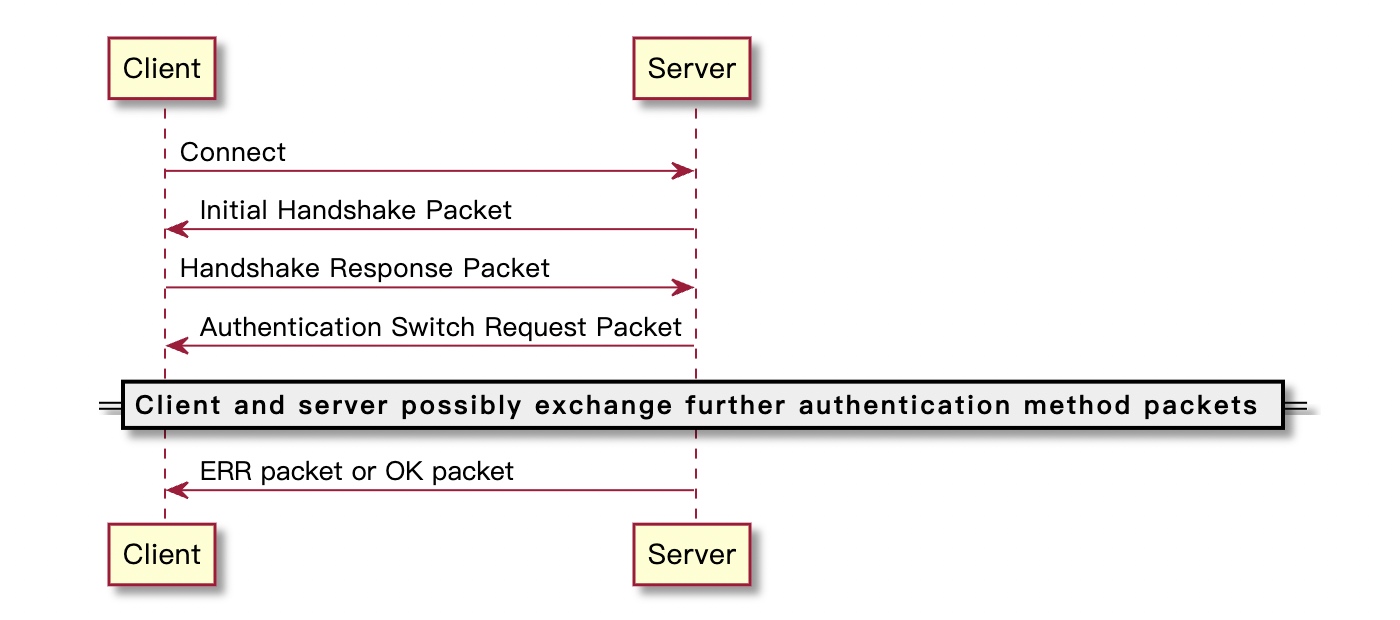
Authentication Plugin配置
Authentication Plugin配置在MySQL的用户表(
mysql.user
)里:其中plugin里存的就是该用户应该使用的身份认证插件,而
authentication_string
则是这个插件需要用来验证身份的相关信息(比如算法、加密轮次、Salt、密码经过加密之后的密文等等),不同的插件存储的信息不同。三种常用的身份认证插件
这里介绍三种常用的插件
mysql_native_password
MySQL 5.6/5.7的默认密码插件一直以来都是
mysql_native_password
。其优点是它支持challenge-response
机制,这是非常快的验证机制,无需在网络中发送实际密码,并且不需要加密的连接。然而,mysql_native_password
依赖于SHA1
算法,但NIST
(美国国家标准与技术研究院)已建议停止使用SHA1
算法,因为SHA1
和其他哈希算法(例如MD5
)已被证明非常容易破解。此外,由于
mysql_native_password
在mysql.user
表中authentication_string
字段存储的是两次哈希SHA1(SHA1(password))
计算的值,也就是说如果两个用户帐户使用相同的密码,那么经过mysql_native_password
转换后在mysql.user
表得到的哈希值相同。尽管有hash值也无法得到实际密码信息,但它仍然告诉这两个用户使用了相同的密码。为了避免这种情况,应该给密码加盐(salt),salt基本上是被用作输入,用于转换用户密码的加密散列函数。由于salt是随机的,即使两个用户使用相同的密码,转换后的最终结果将发生较大的变化。我们可以通过下面的sql来验证
mysql_native_password
的存储的authentication_string
sha256_password
从MySQL 5.6开始支持
sha256_password
认证插件。它使用一个加盐密码(salted password)进行多轮SHA256
哈希(数千轮哈希,暴力破解更难),以确保哈希值转换更安全。然而,它需要要么在安全连接或密码使用RSA秘钥对加密。所以,虽然密码的安全性更强,但安全连接和多轮hash转换需要在认证过程中的时间更长。sha256_password
的authentication_string
总共是67个字节,其中3个$
分隔符内容 | 字节数 | 说明 |
哈希算法 | 1字节 | 5 表示 SHA256 算法、6 表示 SHA512 算法 |
盐(salt) | 20字节 | 用于解决相同密码相同哈希值问题 |
哈希值 | 43字节 | ㅤ |
caching_sha2_password
为了克服这些限制,从MySQL 8.0.3开始,引入了一个新的身份验证插件
caching_sha2_password
。从 MySQL 8.0.4开始,此插件成为MySQL服务器的新默认身份验证插件。caching_sha2_password
尝试一个两全其美的结合,既解决安全性问题又解决性能问题。首先,是
caching_sha2_password
对用户密码的处理,其实主要是sha256_password
的机制:- 使用
SHA2
算法来转换密码。具体来说,它使用SHA256
算法。
- 保存在
authentication_string
列中的哈希值为加盐后的值,由于盐是一个20-byte的随机数,即使两个用户使用相同的密码,转换后的最终结果也将完全不同。
- 为了使使用暴力破解机制更难以猜测密码,在将最终转换存储在
mysql.user
表中之前,对密码和盐进行了 5000轮SHA2
散列。
支持两种操作方式:
- COMPLETE:要求客户端安全地发送实际密码(通过TLS连接或使用RSA密钥对)。服务器生成5000轮哈希,并与mysql.user中存储的值进行比较。
- FAST:允许使用SHA2哈希的进行基于
challenge-response
的身份验证。同时实现高性能和安全性。
caching_sha2_password
的authentication_string
总共是70个字节,其中3个$
分隔符(盐和hash中间没有分隔符)内容 | 字节数 | 说明 |
哈希算法 | 1字节 | 目前仅为 A,表示 SHA256 算法 |
哈希轮转次数 | 3字节 | 目前仅为 005,表示 5*1000=5000 次 |
盐(salt) | 20字节 | 用于解决相同密码相同哈希值问题 |
哈希值 | 43字节 | ㅤ |
sha256_password
和caching_sha2_password
的客户端插件在传递密码时,如果是加密连接,可以直接传输明文密码。如果是非加密连接,必须要用服务端的RSA公钥加密之后再传输。抓包
下面展示几种不同身份认证场景下的抓包信息
用户配置和默认一致
都是mysql_native_password,可以看到,账号密码都正确,认证成功,客户端已经开始正常发送命令了
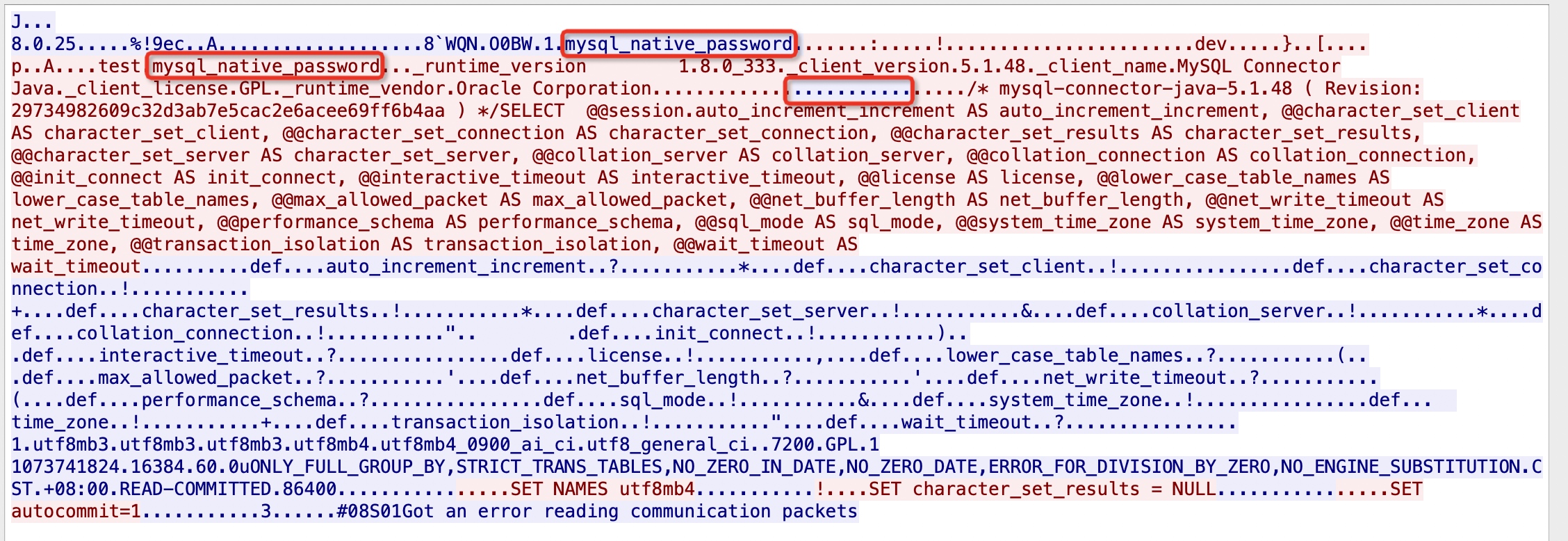
Auth Switch抓包
下面展示两个Auth Switch的抓包
一个是从
caching_sha2_password
切换到sha256_password
,并且可以看到中间还向服务端请求了RSA公钥(这里客户端必须配置allowPublicKeyRetrieval=true
)。当然也可以通过serverRSAPublicKeyFile
来指定RSA公钥的文件路径。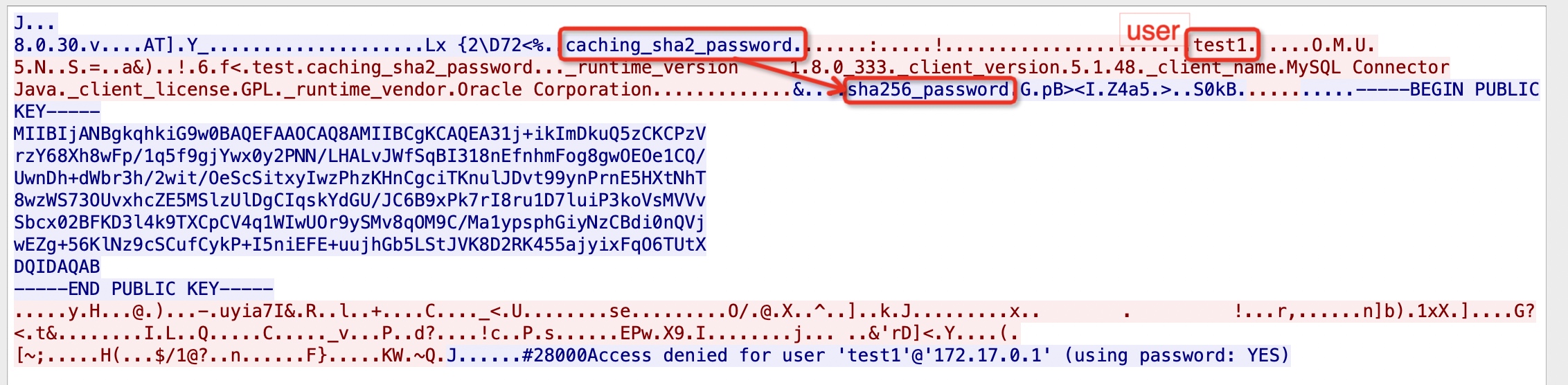
一个是从
caching_sha2_password
切换到mysql_native_password

Auth Switch Request之后的Auth Switch Response,只需要发送加密后的密码信息即可。
allowPublicKeyRetrieval默认值是false,因为打开了之后,可能会存在中间人攻击。
Note that allowPublicKeyRetrieval=True could allow a malicious proxy to perform a MITM attack to get the plaintext password, so it is False by default and must be explicitly enabled.
加密连接
如果双方都想要建立加密连接,那么就要先进入SSL/TLS握手流程:
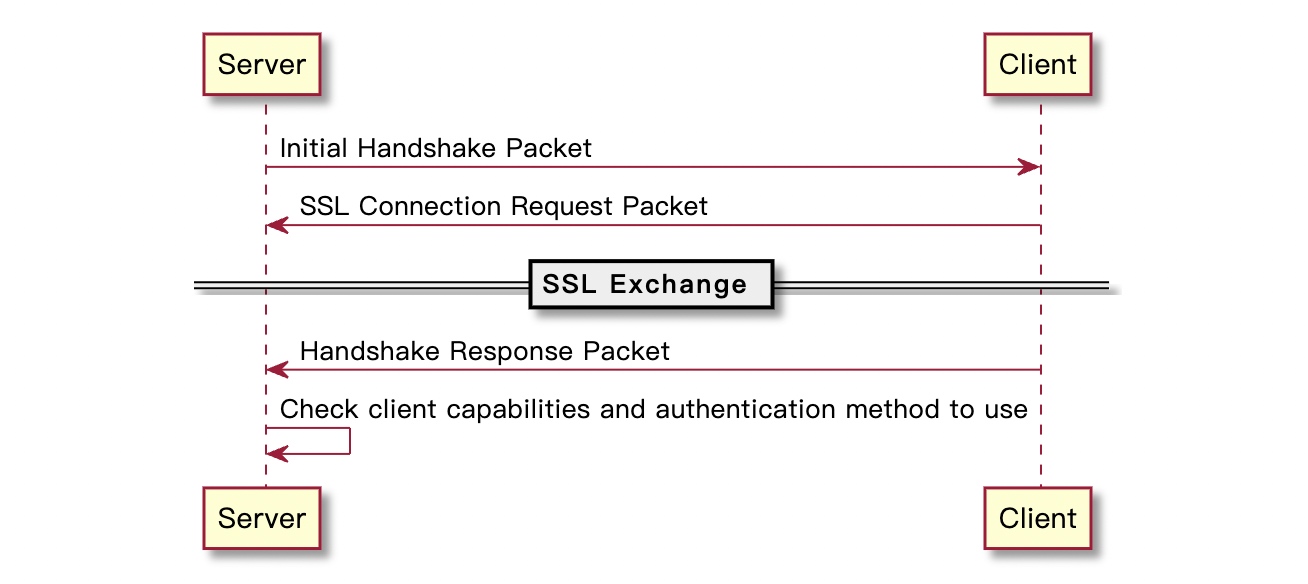
SSL/TLS 握手
简单说一下SSL/TLS的握手过程:
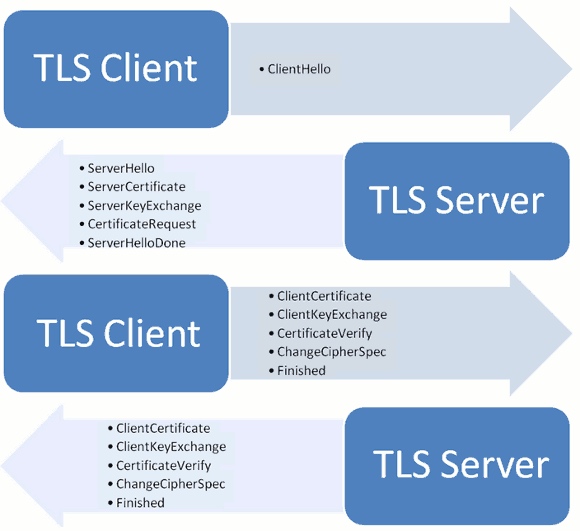
- ClientHello
- 客户端声明自己支持的一些能力(TLS版本/加密方法/压缩方法等)
- 产生一个随机数,稍后用于生成“对话密钥”
- ServerHello
- 确定要用的TLS版本、加密方法等
- 产生一个随机数,稍后用于生成“对话密钥”
- 服务器证书下发
- 客户端回应
- 客户端先验证证书合法
- 再产生一个随机数(pre-master),用于生成“对话密钥”,并用服务端公钥加密
- 编码改变通知,表示随后的信息都将用双方商定的加密方法和密钥发送。
- 客户端握手结束通知,表示客户端的握手阶段已经结束。这一项同时也是前面发送的所有内容的hash值,用来供服务器校验
- 服务端最后回应
- 编码改变通知,表示随后的信息都将用双方商定的加密方法和密钥发送
- 服务器握手结束通知,表示服务器的握手阶段已经结束。这一项同时也是前面发送的所有内容的hash值,用来供客户端校验
其实握手主要就是确定TLS协议版本以及沟通产生对话密钥,用于接下来的加密通信。详细的过程可以阅读阮一峰老师的科普文章——SSL/TLS协议运行机制的概述
关于Sha256PasswordPlugin的理解误区
看了
Sha256PasswordPlugin
客户端插件的源代码,没有找到和Sha256相关的hash算法。反而是:这不是Sha1吗?为什么要叫Sha256呢?
看了同样有人提过这个问题,但是里面也没有人解释,只是说了存在服务端的
authentication_string
的含义。不过看到这段话我突然醒悟:这个客户端的Sha256PasswordPlugin
可能只是为了传输的安全性,而Sha256则是服务端计算authentication_string
时用到的hash算法。正确的流程应该是,客户端通过RSA算法加密之后传到服务端,服务端解密后拿到密码的原值再进行Sha256哈希算法进行计算,计算之后和authentication_string
里的签名部分进行比较。用户不存在时的认证问题
如果登录用户不存在于
mysql.user
表不是应该直接报错么?你可能也是这么想的。但是测试下来事实好像并非如此。下面是两个异常CASE的抓包信息,两次认证的用户都不存在,default_authentication_plugin
配置的是caching_sha2_password
。但是两次都走了Auth Switch流程,并且不存在的两个用户,服务端切换到了不同的身份认证插件。尝试了
flush PRIVILEGES;
或者重启MySQL,也没有效果,应该和caching_sha2_password
的缓存无关异常CASE1
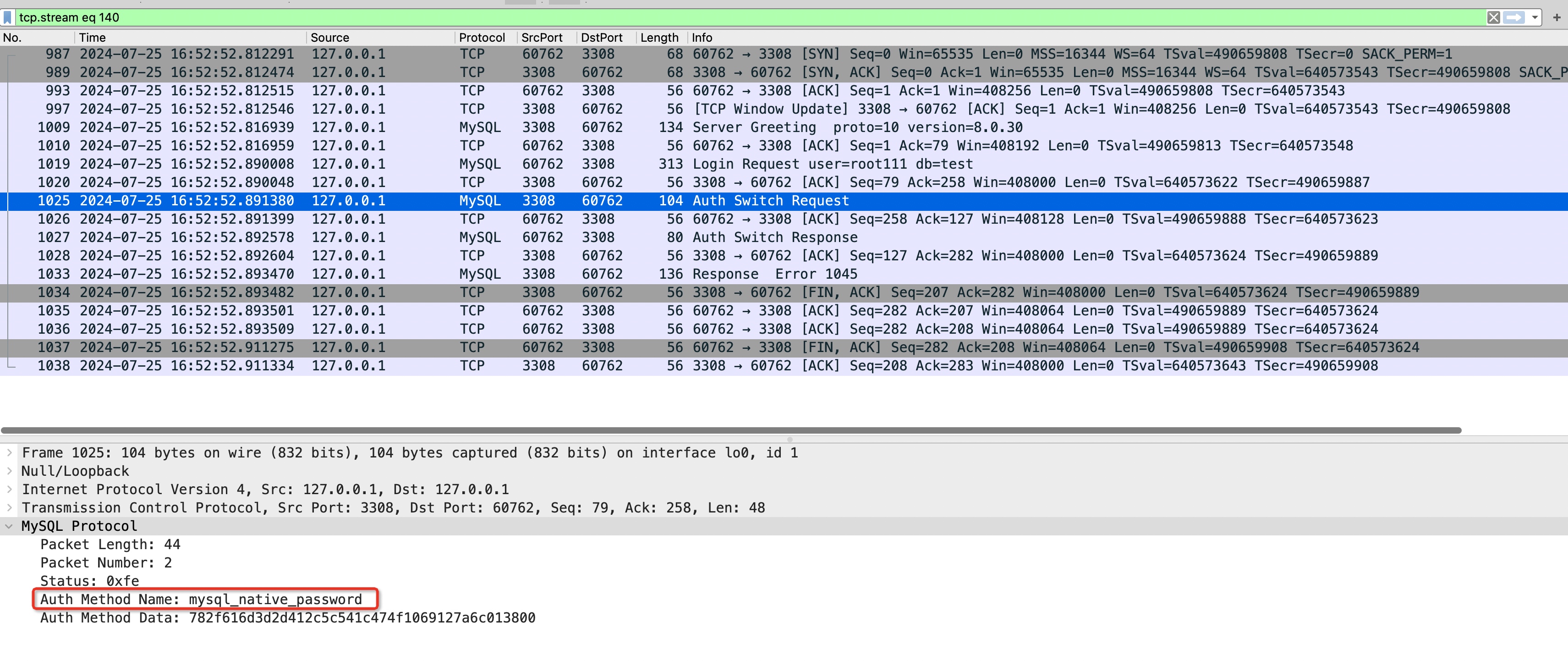
异常CASE2
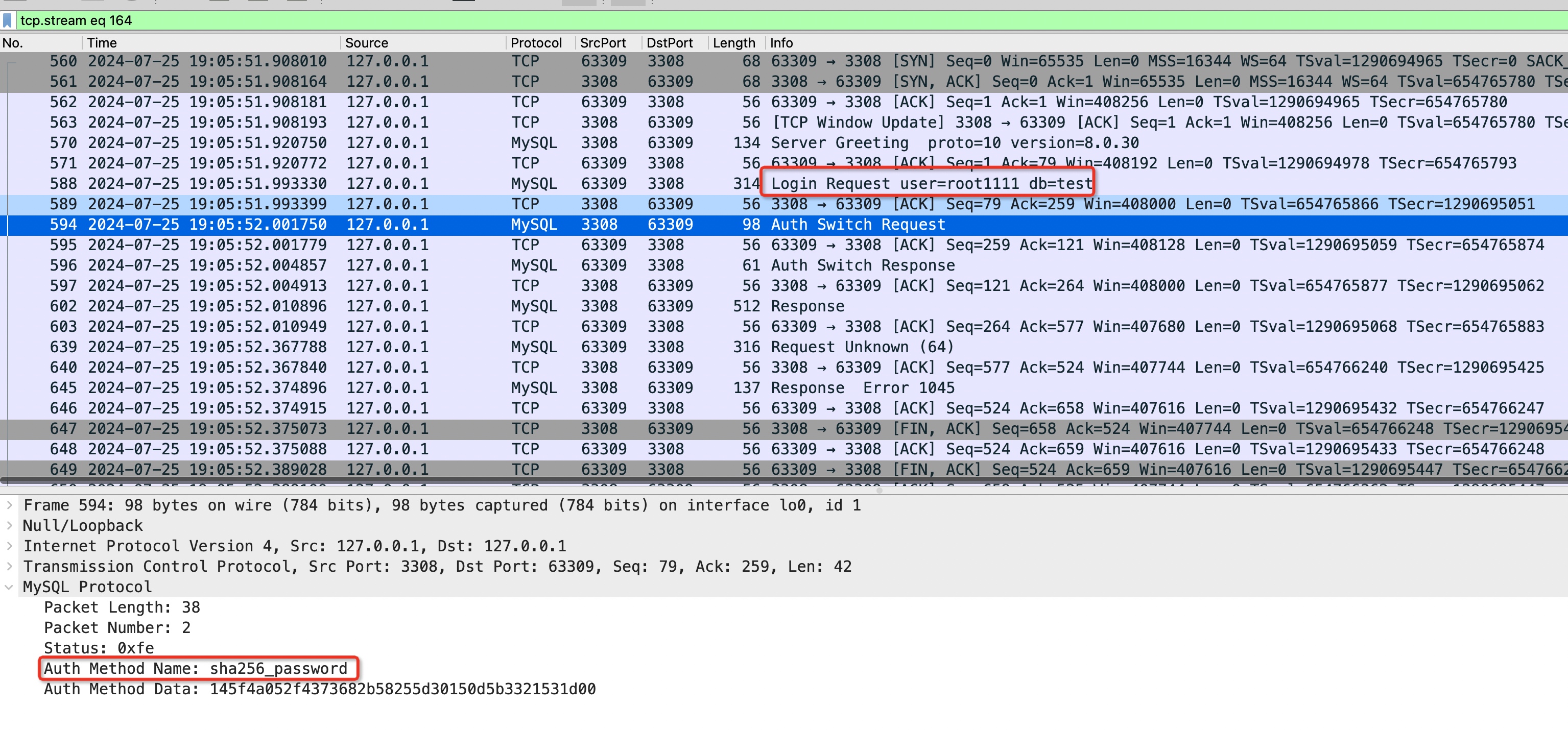
参考
- Author:黑微狗
- URL:https://blog.hwgzhu.com/article/mysql-connection-phase
- Copyright:All articles in this blog, except for special statements, adopt BY-NC-SA agreement. Please indicate the source!
Relate Posts